In the changing world of web development, user experience is everything. Gone are the days of static web pages; today’s users expect dynamic, interactive applications. Angular, a powerful front-end framework, empowers developers to create seamless and engaging web experiences through its routing and navigation capabilities.
In this blog, we’ll explore the basics of Angular routing and navigation and see how they contribute to creating dynamic web applications.
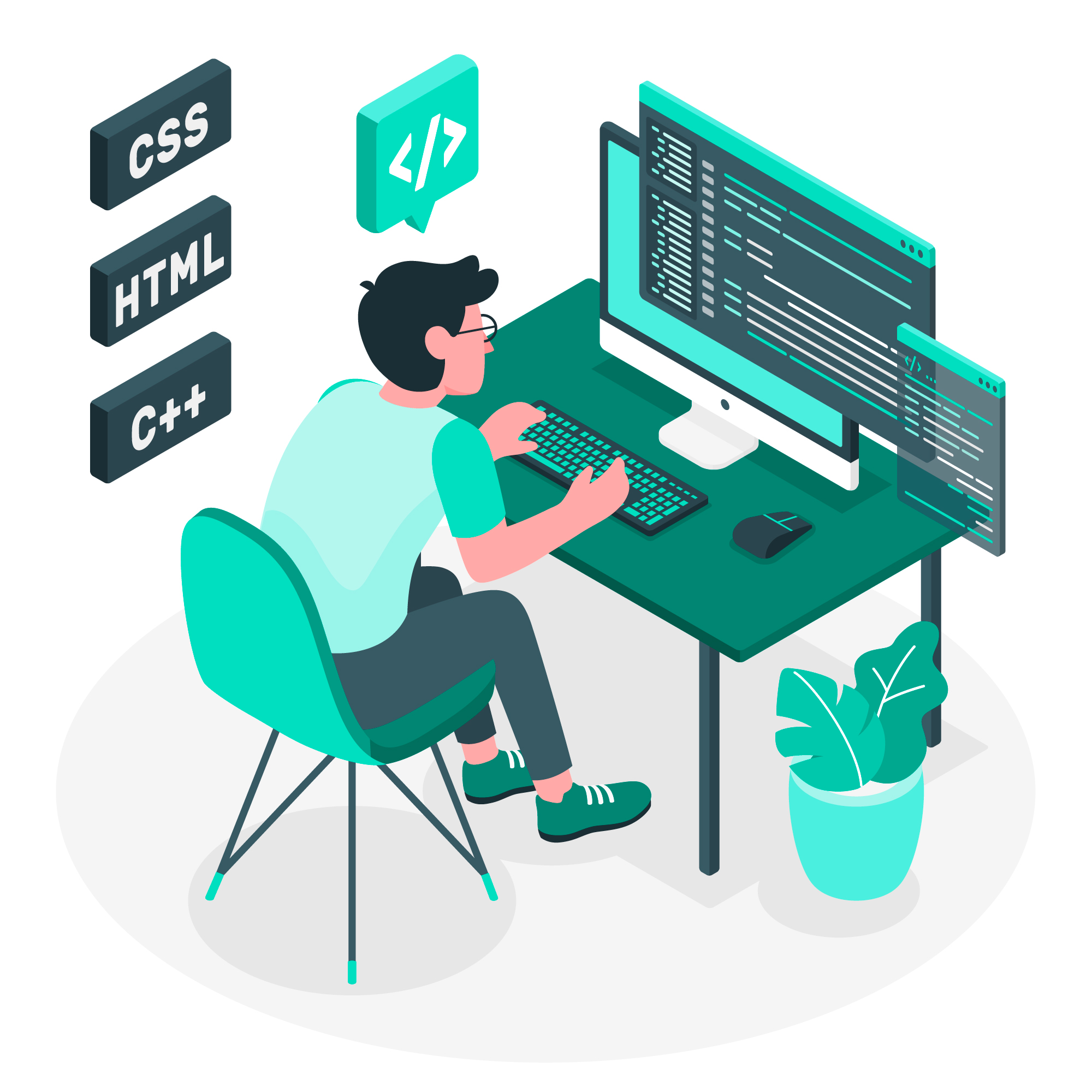
Understanding Angular Routing
Routing in Angular is like following a map to find your destination. Just as a map guides you to different locations, routing directs users to various parts of your application based on the URL or user interactions. It’s the core mechanism behind creating single-page applications (SPAs), where content changes without requiring full page reloads, resulting in faster, smoother experiences. Angular uses the concept of a “single page application” (SPA) where the initial page is loaded, and then, as the user interacts with the application, the content dynamically changes without requiring a full page reload. This approach significantly improves performance and enhances the user experience.Setting Up Routes in Angular:
Setting up routes in Angular is straightforward. You define routes, associate them with components, and specify how components should display within the application. Here’s a simple example:This code sets up two routes: one for the Home component and another for the About component.
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home.component';
import { AboutComponent } from './about.component';
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppModule { }
Navigating Between Routes:
The Angular offers different ways to move between routes. You can use the routerLink directive in templates or programmatically navigate using the Angular Router service.
1. Using routerLink:
2. Programmatically navigating:
import { Router } from '@angular/router';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['./home.component.css']
})
export class HomeComponent {
constructor(private router: Router) {}
navigateToAbout() {
this.router.navigate(['/about']);
}
}
Dynamic Route Parameters and Data:
In AngularĀ you can pass parameters through the URL, making routes more dynamic. You can define parameters in the route path and access them in the component using the ActivatedRoute service.
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'user/:id', component: UserProfileComponent },
];
// user-profile.component.ts
import { ActivatedRoute } from '@angular/router';
export class UserProfileComponent {
constructor(private route: ActivatedRoute) {
this.route.params.subscribe(params => {
const userId = params['id'];
// Fetch user data based on userId
});
}
}
Child Routes:
For complex component hierarchies, Angular allows creating child routes. These routes are rendered within the context of a parent component.const routes: Routes = [
{ path: '', component: HomeComponent, children: [
{ path: 'child', component: ChildComponent },
{ path: 'another-child', component: AnotherChildComponent }
]},
];
Summary
Routing and navigation are vital for building dynamic web experiences. With Agular’s robust routing features, you can create single-page applications with smooth transitions between components. Embrace Agular’s routing capabilities, and elevate your web application to new heights of interactivity and user satisfaction.
For more information about the Angular routing please visit this link
Angular React technologies plays crucial role in the web development. For more information please visit the Patterns7 Monolithic enterprise software to Microservices based architecture
Recent Comments